The approach for authorization in Laravel is quite simple and easy. In this blog, we will talk about LARAVEL gates and policies for authorization. After introducing the terms like authorization, authentication, gates, and policies, we will implement the concepts with an example. But before that, let’s understand the terms we will use in this blog of LARAVEL authentication.
WHAT IS LARAVEL AUTHENTICATION
?
Laravel authentication is used to identify the user credentials and is managed by sessions that take the input like email or username and password for user identification.
HOW TO AUTHORIZE
?
- Closure based approach
- https://xd.adobe.com/view/7bdcda5e-de4f-449e-b4b9-d5a578effa33-2e8f/
The closure-based approach of authorization is called Gates, and the class-based approach is called policy. In this document, we will tell you to authorize using both gates and closure-based routes.
WHAT IS THE DIFFERENCE
BETWEEN AUTHORIZATION
AND AUTHENTICATION
:
As the name suggests, authentication confirms the identity of the users or makes sure they are what they claim to be. Meaning, a user can only work on those modules and actions which have been allowed to them.
Both authentication and authorization are distinct security processes in the world of identity and access management (IAM) in Laravel 8. However, authorization permits the right users to access the resources. We will talk about this in detail in a later part of the document.
GATES VS.
POLICIES IN LARAVEL
:
Laravel Gates and policies are like routes and controllers, respectively. Gates allows users to define an authorization using a simple closure-based approach. If you want an action unrelated to any specific model, gates are perfect to use.
Gates must have a user instance as its first argument and may optionally have additional arguments as a relevant Eloquent model. So, now you know how to use gates to authorize actions in your Laravel application.
The next section is all about updating an article:
<?php
use App\Models\Article;
use App\Models\User;
use Illuminate\Support\Facades\Gate;
/**
* Register any authentication / authorization services.
*
* @return void
*/
public function boot()
{
$this->registerPolicies();
Gate::define('update-article', function (User $user, Article $article) {
return $user->id === $article->user_id;
});
}
The Gates are defined in the boot method of the App\Providers\AuthServiceProvider class, and it uses the Gate facade.
Let’s understand this with an example, where a user can update an article Model App\Models\Article; the gate will do this by comparing the user’s id against the user_id that created the article. Please see the example below:
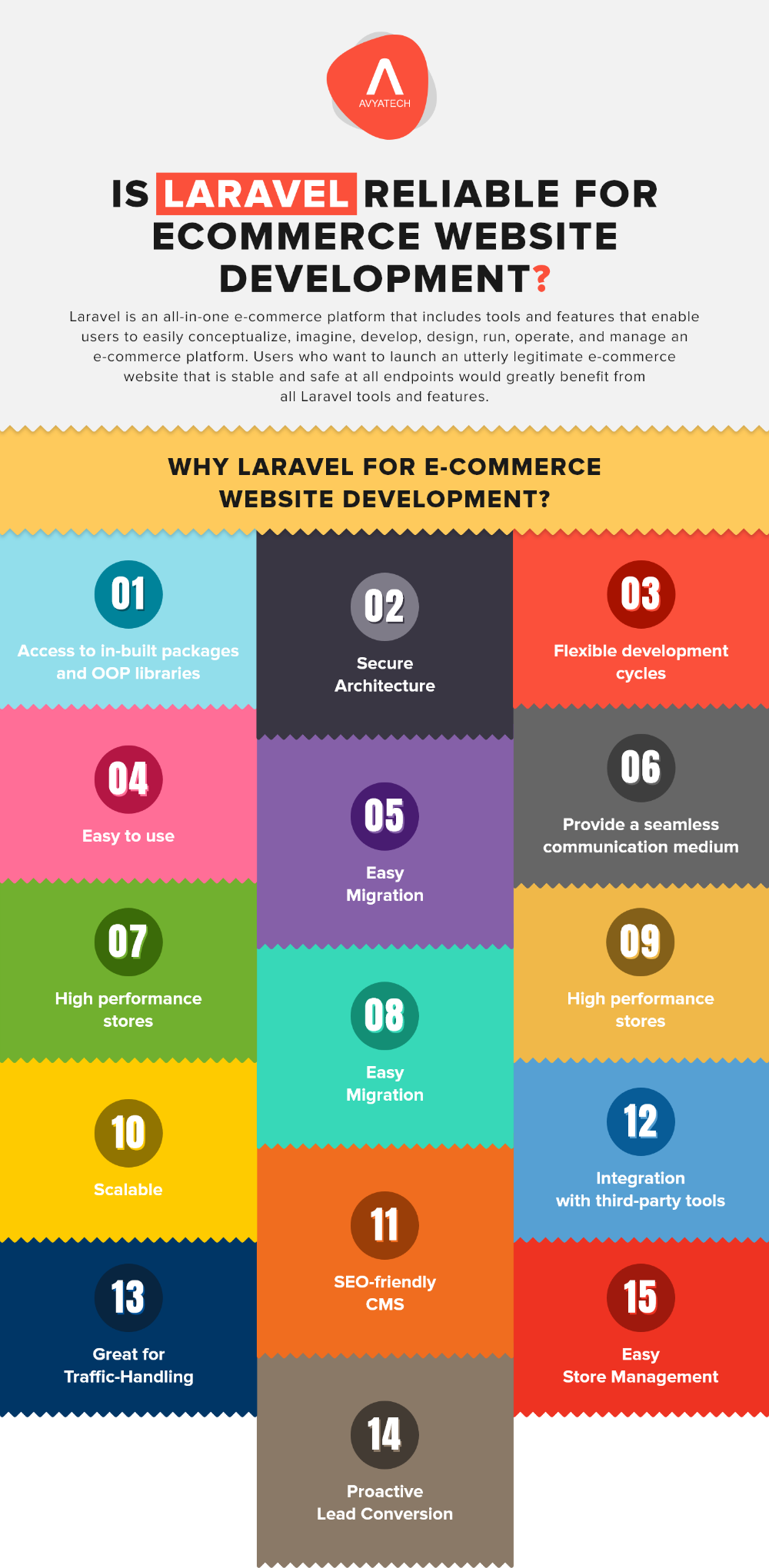
WHAT IS THE DIFFERENCE
BETWEEN AUTHORIZATION
AND AUTHENTICATION
:
The Gates are defined in the boot method of the App\Providers\AuthServiceProvider class, and it uses the Gate facade.

POLICIES
The policies are a way to logically organise authorization for a particular model or resource.
- App\Modeils|Article model
- and we can have an App\Policies\ArticlePolicy to authorize the user actions.
- To generate a policy we can use the make: policy Artisan command, it will be created here app/Policies/ArticlePolicy.php:
- php artisan make: policy ArticlePolicy
- // the above will generate an empty policy class.
- php artisan make:policy ArticlePolicy –model=Article
- // the above will generate a class with example policy methods related to viewing, creating, updating, and deleting the resource.
HOW TO AUTHORIZE
ACTIONS IN LARAVEL 8
We can authorize actions using gates in Laravel 8. You can use “allows” or “denies” methods profiled by the Gate facade. We don’t need to pass the currently authenticated user to these methods, laravel will automatically pass the user into the gate closure. We need to call gate authorization methods within the application controllers before performing an action that requires authorization.
Please see the example below:
<?php
namespace App\Http\Controllers;
use App\Http\Controllers\Controller;
use App\Models\Article;
use Illuminate\Http\Request;
use Illuminate\Support\Facades\Gate;
class PostController extends Controller
{
public function update(Request $request, Article $article)
{
if (! Gate::allows('update-article', $article)) {
abort(403);
}
// Update the article code here...
}
}
The above code check for the user if he is allowed to perform the action, And if you want to determine if a user other then the currently authenticated user is authorized for the action then need to use for user method on the Gate facade: